Java,J2ee,Websphere/Weblogic Portal,Design Patterns,Advanced Alogorithms, Networking...
Tuesday, July 27, 2010
Client code accessing a webservice
Wednesday, July 14, 2010
Jasper Reports with iReport Editor.
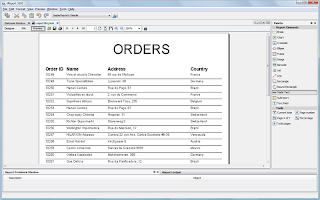
Reports Creation made simple in Java.
1. Creating a data source or a database connection used to fill the report.
2. Designing the report, including the layout of its elements and parameters to represent the data.
3. Runing the report, which includes compiling the JRXML source file in a Jasper file and filling in the data for export or onscreen display.
When you design and preview a report in iReport, iReport produces a Jasper file. This file is all you need in your Java application to generate and display the report (in addition to JasperReports, of course).
The report execution is performed by the JasperReports library, so you have to include all the required jars in your application. Which jars? If you do not have a problem shipping a lot of jars, you can include all the jars provided in JasperReports. However JasperReports includes many jars that you may not need, such as ones to create barcodes, the ones used to handle XML files (as long as you don't use an XML datasource), and ant.jar and servlet.jar, which are provided to compile the source code. Other optional jars are hsqldb.jar that is shipped to run the sample database and bsh-2.0b4.jar used to compile and run the reports using Beanshell. Other jars may be already included in your environment, for example Spring, Apache Commons Logging, and Mondrian (an OLAP database server), among others.
Here is the complete list of jars shipped with JasperReports 3.6.0 (the list is subject to change in future versions):
ant-1.7.1.jar antlr-2.7.5.jar barbecue-1.5-beta1.jar barcode4j-2.0.jar batik-anim.jar batik-awt-util.jar batik-bridge.jar batik-css.jar batik-dom.jar batik-ext.jar batik-gvt.jar batik-parser.jar batik-script.jar batik-svg-dom.jar batik-svggen.jar batik-util.jar | batik-xml.jar bcel-5.2.jar bsh-2.0b4.jar commons-beanutils-1.8.0.jar commons-collections-2.1.1.jar commons-digester-1.7.jar commons-javaflow-20060411.jar commons-logging-1.0.4.jar groovy-all-1.5.5.jar hibernate3.jar hsqldb-1.8.0-10.jar iText-2.1.0.jar jasperreports-3.6.0.jar jaxen-1.1.1.jar jcommon-1.0.15.jar jdt-compiler-3.1.1.jar | jfreechart-1.0.12.jar jpa.jar jxl-2.6.jar log4j-1.2.15.jar mondrian-3.1.1.12687.jar png-encoder-1.5.jar poi-3.2-FINAL-20081019.jar rhino-1.7R1.jar saaj-api-1.3.jar servlet.jar spring-beans-2.5.5.jar spring-core-2.5.5.jar xalan-2.6.0.jar xercesImpl-2.7.0.jar xml-apis-ext.jar xml-apis.jar |
Here is a simple application to create a PDF from a Jasper file using an empty data source. An empty data source is a data source that by default has a single record and for which you can define any field name to use in the report, but its value will always be null. It is a perfect data source to test a very simple report displaying just the label "Hello World!" For real applications, you will probably use a JDBC connection, a collection of JavaBeans, or a Hibernate session to provide data for the report. But for the aim of this tutorial, the empty data source is good enough.
import net.sf.jasperreports.engine.*;
import net.sf.jasperreports.engine.export.*;
import java.util.*;
public class JasperTest {
public static void main(String[] args) {
String fileName = "test.jasper";
String outFileName = "test.pdf";
HashMap hm = new HashMap();
try {
// Fill the report using an empty data source
JasperPrint print = JasperFillManager.fillReport(fileName, hm, new JREmptyDataSource());
// Create a PDF exporter
JRExporter exporter = new JRPdfExporter();
// Configure the exporter (set output file name and print object)
exporter.setParameter(JRExporterParameter.OUTPUT_FILE_NAME, outFileName);
exporter.setParameter(JRExporterParameter.JASPER_PRINT, print);
// Export the PDF file
exporter.exportReport();
} catch (JRException e) {
e.printStackTrace();
System.exit(1);
} catch (Exception e) {
e.printStackTrace();
System.exit(1);
} } }
The most important line of code in this example is line 13. The class JasperFillManager provides several static methods to fill a Jasper file, using a data source, a connection, or even nothing. The result of the call to the method fillReport() is the special JasperPrint object which contains an in-memory representation of the report. This object can be previewed by using the JasperReports Viewer, a convenient Swing component to display the report on screen (it's the same component used by the iReport preview). As in this example, the JasperPrint object can also be passed to an exporter to generate a final document in a specific format.
On line 16, the JRPdfExporter is instantiated and later configured by setting the JasperPrint and the output file. The exportReport() method of the exporter does the rest, creating the file test.pdf.
In this sample we stored the result in a file. In other situations, such as in web applications, it is much more convenient to send the exported bytes directly to the browser. This can be done by setting the OUTPUT_STREAM parameter of the exporter to the output stream of the web application. There are many other export options, some of them common to all export formats (such as the range of pages to export), others specific of the exporter implementation.
Friday, July 2, 2010
Concurrency Control Issue- "Optimistic Update Access Intent"
In one of our project we used EJB’s and It is not working when migrated from Websphere Server 5.1 to Websphere server 7.0.
It started throwing deadlock exceptions when multiple users clicks the save button at the same time.
This is happening because two sessions going to the same functions and the function is under Transaction Attribute “Required”, which means the method should be under transactional context.
If a calling method is under transactional context then this method follows the same transaction, if is not under transactional context than websphere container intiates a new transaction. Here the problem is not with the transactional attribute. The problem is with “Concurrency Control” Issue. Both sessions trying to update the same table then they are getting locks on the table and after updating the tables they have to load the case from the database. So both are trying to read the database and while reading they are locking the table’s again (which is not required to lock the table while reading—we wanted a dirty read). This is happening because by default websphere server uses “Pessimistic Update” Access Intent on all Entitiy Beans. Pessimistic Update locks the table even for reading also by issuing “SELECT…..FOR UPDATE” Statement.
Changing Access Intent from “Pessimistic Update” to “Optimistic Update” resolved the issue. In Optimistic Update it doesn’t lock on table for reading and it issues simple “SELECT” statement.
Finally the deadlock issue is Resolved. Finding the issue was tough than the solution.
Here goes log of Dead Lock stack Trace.
*** MODULE NAME:(JDBC Thin Client) 2010-06-24 13:09:17.861
*** SERVICE NAME:(SYS$USERS) 2010-06-24 13:09:17.861
*** SESSION ID:(901.1366) 2010-06-24 13:09:17.861
DEADLOCK DETECTED ( ORA-00060 )
[Transaction Deadlock]
The following deadlock is not an ORACLE error. It is a
deadlock due to user error in the design of an application
or from issuing incorrect ad-hoc SQL. The following
information may aid in determining the deadlock:
Deadlock graph:
---------Blocker(s)-------- ---------Waiter(s)---------
Resource Name process session holds waits process session holds waits
TX-00060012-0003e77d 475 901 X 401 2127 X
TX-0002000f-000521da 401 2127 X 475 901 X
session 901: DID 0001-01DB-00000026 session 2127: DID 0001-0191-0000001C
session 2127: DID 0001-0191-0000001C session 901: DID 0001-01DB-00000026
Rows waited on:
Session 2127: obj - rowid = 000139A5 - AAATmlAAQAAAacCAAf
(dictionary objn - 80293, file - 16, block - 108290, slot - 31)
Session 901: obj - rowid = 000139A5 - AAATmlAAQAAAbBDAA3
(dictionary objn - 80293, file - 16, block - 110659, slot - 55)
Information on the OTHER waiting sessions:
Session 2127:
pid=401 serial=1922 audsid=10835401 user: 7626/ASAPS_WEB
O/S info: user: wasadm, term: unknown, ospid: 1234, machine: surya7.dss.state.
va.us
program: JDBC Thin Client
application name: JDBC Thin Client, hash value=2546894660
Current SQL Statement:
SELECT T1.AS_UAI_QUESTION_ID, T1.QUESTION_TXT, T1.QUESTION_DESC, T1.UAI_LOCATOR, T1.QUESTION_ORDER, T1.LST_UPDT_ID, T1.LST_UPDT_DT FROM OASIS.TAS_UAI_QUESTI
ON T1 WHERE T1.AS_UAI_QUESTION_ID = :1 FOR UPDATE
End of information on OTHER waiting sessions.
Current SQL statement for this session:
SELECT T1.AS_UAI_QUESTION_ID, T1.QUESTION_TXT, T1.QUESTION_DESC, T1.UAI_LOCATOR, T1.QUESTION_ORDER, T1.LST_UPDT_ID, T1.LST_UPDT_DT FROM OASIS.TAS_UAI_QUESTION
T1 WHERE T1.AS_UAI_QUESTION_ID = :1 FOR UPDATE